Understanding Swift: Basics for Beginners
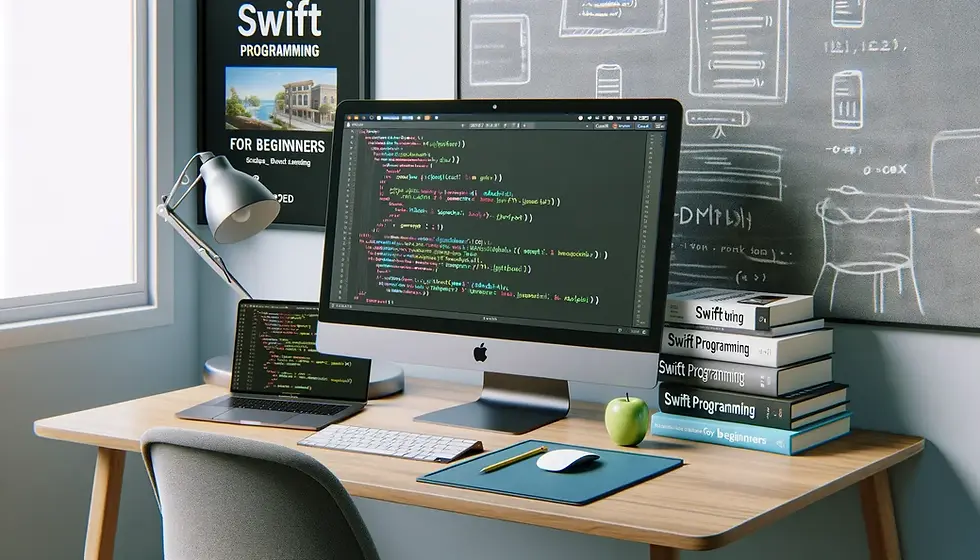
Swift is an advanced, contemporary programming language created by Apple to facilitate application development across its various platforms, including iOS, macOS, watchOS, and tvOS. Launched in 2014, Swift is engineered to be intuitive for beginners yet robust enough for intricate tasks. It emphasizes type-safety, assisting you in crafting more understandable and dependable code, thereby reducing errors. Swift has rapidly risen as one of the quickest expanding languages historically, attributed to its straightforwardness, efficiency, and scalability. This piece delves into Swift’s basic elements, providing novices with insights into its syntax, fundamental principles, and recommended practices.
Configuring a Swift Development Setup
Initiating Swift development requires configuring Xcode on a Mac, as it consolidates all necessary development utilities.
Xcode offers a source editor, a debugger, and tools for designing interfaces, all crucial for Swift development.
Users of Windows or Linux can engage cloud-based IDEs or Swift compilers for developing Swift applications.
An adequately prepared environment is pivotal for effective coding and testing of applications.
Acquainting oneself with Xcode’s capabilities substantially boosts your proficiency in Swift coding.
To embark on Swift development, setting up an environment is essential, and Xcode is the primary tool, available solely on Mac systems. Xcode amalgamates all vital tools, including a source editor, debugger, and interface designers, among others. After installing Xcode from the Mac App Store, initiating new Swift projects and commencing coding is straightforward, utilizing its robust functionalities. For non-Mac users, alternatives include direct usage of a Swift compiler or cloud-based IDEs that support Swift. Setting this environment is your initial step towards Swift mastery.
Syntax Basics and Structure
The syntax of Swift is designed to be succinct, aiding in the readability and writability of your code. Variables are mutable and declared with var, while constants are immutable and declared with let. The language enforces strict data types but allows the compiler to infer types based on initial values. Unlike many languages, Swift does not require semicolons at the end of statements, unless you’re placing multiple statements on a single line. Grasping these syntax and structure basics is vital for crafting clear and efficient Swift code.
Flow Control
Swift’s flow control capabilities include familiar constructs like if, else, switch, and various loops (for-in, while, repeat-while).
The switch statement is adaptable, supporting an extensive range of types and complex conditions without the need for breaks.
Looping mechanisms in Swift are robust, providing effective ways to traverse elements in collections such as arrays and dictionaries.
Proper employment of flow control statements is crucial for guiding program execution and managing various scenarios.
Learning Swift’s flow control is fundamental to developing dynamic and responsive applications.
Flow control in Swift enables the crafting of intricate conditional and looping structures using standard statements such as if, else, switch, and different types of loops, including for-in, while, and repeat-while. Swift’s flow control tools are potent, with switch statements that accommodate a variety of data types and complex conditions without necessitating explicit break statements in each case. These features allow developers to write more expressive and comprehensive code efficiently handling different scenarios. Mastery of flow control is essential for directing the execution paths of your programs.
Functions
Functions in Swift are designated with the func keyword and can incorporate multiple parameters and return types.
Swift supports default parameter values, enhancing the flexibility and adaptability of functions to various scenarios.
Functions can return multiple values using tuples, which enhances complex data manipulation capabilities.
Functions in Swift are first-class entities that can be passed and utilized like any other type, offering significant versatility.
Comprehending and employing functions is crucial for effectively organizing and reusing code in Swift applications.
Functions in Swift are foundational elements that can be passed around in your code as any other type. They are declared with the func keyword, followed by their name and a parameter list in parentheses. Swift functions can return a single value or multiple values using tuples. Moreover, Swift accommodates functions with default parameter values, adding to their versatility. Understanding and using functions efficiently is fundamental in Swift, as they facilitate the organization and reuse of code across your applications.
Optionals
Optionals are a distinctive feature in Swift, designed to explicitly handle the absence of a value.
Declared by appending a ? to any type, optionals signify that a variable may contain either a value or nil.
They are crucial for crafting safe code, compelling developers to consciously handle nil values to avert runtime errors.
Optional binding and chaining are techniques employed to access optional values safely.
Mastery of optionals is essential for crafting robust Swift applications that gracefully manage missing or incomplete data.
Optionals are a unique feature in Swift, addressing the absence of a value for any type. An optional is declared by appending a ? to the type name, indicating that the variable can hold either a value or nil, representing no value at all. Optionals are vital because they compel you to handle nil values safely and explicitly, thus preventing common runtime errors found in other languages. Using optionals effectively can help you write safer and more reliable code by ensuring that you consider cases where data might not be available. Swift provides several mechanisms, like optional binding and optional chaining, to work with optionals more conveniently.
Collections
Swift offers three primary collection types: arrays, sets, and dictionaries, each serving distinct data organization needs.
Arrays store ordered collections, sets manage unique items without an order, and dictionaries store items as key-value pairs.
Collections in Swift are type-safe, ensuring the consistency and correctness of the data they store.
Swift provides extensive methods for manipulating collections, such as sorting, filtering, and mapping data.
Proficiency with collections is crucial for managing grouped data efficiently in Swift applications.
Swift provides various collection types, each tailored for different programming needs, including arrays, sets, and dictionaries. Arrays maintain items in an ordered sequence, sets store unique items without any order, and dictionaries organize items as key-value pairs. Each collection type is fully integrated with Swift's type system, ensuring they are both type-safe and capable of holding any specific type of values you specify. Manipulating these collections is straightforward with Swift's comprehensive array of methods and properties, making tasks like sorting, filtering, and transforming collections efficient and concise.
Classes and Structures
Swift enables developers to define complex data models using classes and structures.
Classes are reference types, passed by reference, whereas structures are value types and passed by value.
Deciding when to use classes or structures depends on how data needs to be managed and shared within your application.
Both can possess properties and methods, but only classes support inheritance.
Knowledge of classes and structures is fundamental to designing efficient data models in Swift.
Swift supports both classes and structures, which are utilized to create complex data models in your applications. Although similar in many ways, such as defining properties and methods, there are crucial differences: classes are reference types and structures are value types. This distinction affects how they are stored and copied when you pass them around in your code. Understanding the differences between classes and structures aids in deciding which one to use based on the functionality required for your data structures.
Inheritance and Protocols
Inheritance enables Swift classes to inherit properties, methods, and other characteristics from a base class.
Protocols in Swift define a blueprint of methods and properties that classes, structures, or enumerations can adopt.
Using protocols fosters a flexible and modular coding approach, known as protocol-oriented programming.
Inheritance and protocols are central to leveraging Swift’s object-oriented and protocol-oriented programming features effectively.
Comprehending these concepts is key to constructing extensible and maintainable code in Swift.
In Swift, classes can inherit from other classes, allowing you to create a new class that inherits properties, methods, and other characteristics from an existing class. Unlike classes, structures do not support inheritance. However, both classes and structures can conform to protocols. Protocols define a blueprint of methods, properties, and other requirements that suit a particular piece of functionality. Employing inheritance and protocols efficiently allows for more reusable, maintainable, and modular code in Swift applications.
Error Handling
Swift offers robust error handling capabilities that enable developers to catch and manage recoverable errors explicitly.
Errors are defined using types that conform to the Error protocol, and are managed using throws, do, try, and catch keywords.
This structured approach to error handling aids in maintaining program stability and preventing crashes due to unhandled errors.
Learning to implement effective error handling is essential for building reliable and user-friendly applications in Swift.
Swift provides strong error handling capabilities that allow developers to address recoverable errors elegantly. Functions and methods can throw errors, which are declared by the throws keyword and managed using do-catch blocks. This approach ensures that errors are handled explicitly, making the programs more robust and stable. Swift's error handling model aids in preventing crashes by addressing issues at runtime in a controlled manner, thus enhancing the overall reliability of your applications.
Debugging and Testing
Xcode includes sophisticated tools for debugging Swift code, such as breakpoints and an interactive console.
Automated testing via unit tests and UI tests in Xcode helps ensure that your code functions as intended through iterative development cycles.
Effective debugging and testing practices are crucial for developing bug-free and high-quality Swift applications.
Familiarity with Xcode’s debugging and testing tools is invaluable for maintaining and enhancing code quality over time.
Effective debugging and testing are crucial for developing reliable and bug-free applications. Xcode, Swift's primary IDE, includes comprehensive tools for debugging, like breakpoints, an interactive console, and a memory graph debugger. Additionally, Xcode supports unit and UI testing, allowing developers to automate the testing of their code systematically. These tools aid in identifying and resolving bugs more quickly and effortlessly, making your Swift development smoother and more productive.
Memory Management
Swift uses Automatic Reference Counting (ARC) to manage memory, automatically deallocating objects that are no longer necessary.
Developers must be mindful of and manage strong reference cycles, particularly in closures and relationships between class instances.
Tools such as weak and unowned references assist in managing memory within your applications effectively.
Proper memory management is critical for optimizing performance and preventing memory leaks in Swift applications.
Swift uses Automatic Reference Counting (ARC) to manage the application’s memory usage. ARC automatically frees up memory used by class instances when they are no longer necessary. However, developers need to be cautious of strong reference cycles, which can lead to memory leaks. Swift provides several tools, including weak and unowned references, to help developers manage memory effectively in their applications. Understanding and applying these concepts is crucial for building efficient and memory-safe applications.
Advanced Topics
As developers gain proficiency, they may explore advanced Swift topics like concurrency, generics, and custom operators.
These advanced features allow for more sophisticated coding techniques and can help solve more complex problems.
Staying updated with the latest Swift features and best practices is important for professional growth and effective app development.
As developers become more comfortable with the basics of Swift, they may explore more advanced topics, such as concurrency, generics, and advanced data structures. Swift's modern features support complex tasks like asynchronous programming and creating custom generic types. These advanced concepts can help solve more specific problems and enhance the performance and capability of applications.
Best Practices and Tips
Utilizing let extensively for immutability, embracing protocol-oriented programming, and writing comprehensive unit tests are among the best practices in Swift development.
Regularly refactoring code and keeping up with the latest Swift updates can significantly enhance code quality and application performance.
Continuous learning and application of Swift best practices are key to becoming a proficient Swift developer.
Adopting best practices in Swift development is essential for writing clean, efficient, and maintainable code. Some key practices include using constants (let) wherever possible, preferring protocols and generics to enhance flexibility and reusability, and embracing Swift's powerful standard library. Additionally, regularly writing and running tests can ensure your code behaves as expected even as you make changes or add new features.
Conclusion
Swift is an exciting and versatile language that offers a lot for both beginners and seasoned developers. By mastering its fundamental concepts and exploring its advanced capabilities, you can build a wide range of applications for Apple's platforms. The combination of powerful language features, a supportive developer ecosystem, and robust performance makes Swift a top choice for new developers entering the world of software development. Continue learning and experimenting with Swift to build your expertise and craft impressive applications.
This is your Feature section paragraph. Use this space to present specific credentials, benefits or special features you offer.Velo Code Solution This is your Feature section specific credentials, benefits or special features you offer. Velo Code Solution This is
More Ios app Features
How to Get a Refund from Apple: The Ultimate Guide
Learn how to navigate Apple's refund process with ease. This comprehensive guide covers everything from refund eligibility to the steps for requesting refunds on digital purchases, subscriptions, and hardware. Understand Apple's refund policies, explore available options, and discover how to use Apple’s website and support app for a seamless experience. Whether it's an accidental purchase or a faulty product, this manual equips you with the knowledge to secure your refund quickly and efficiently
iOS Development: Tools and Technologies
Explore the latest tools and technologies for iOS development in this comprehensive article. From Xcode and Swift to SwiftUI and Combine, discover the essential tools and frameworks used by iOS developers today. Whether you're a beginner or an experienced developer, this guide offers insights into the tools and technologies shaping the world of iOS app development
Setting Up Your iOS Development Environment
Get started with iOS development by setting up your development environment with this guide. Learn how to install Xcode, set up simulators, and configure your workspace for efficient iOS app development. Whether you're a novice or a seasoned developer, this tutorial will help you establish a solid foundation for building iOS apps